Use native unicode emojis
- gl_emoji for falling back to image/css-sprite when the browser doesn't support an emoji - Markdown rendering (Banzai filter) - Autocomplete - Award emoji menu - Perceived perf - Immediate response because we now build client-side - Update `digests.json` generation in gemojione rake task to be more useful and include `unicodeVersion` MR: !9437 See issues - #26371 - #27250 - #22474
Showing
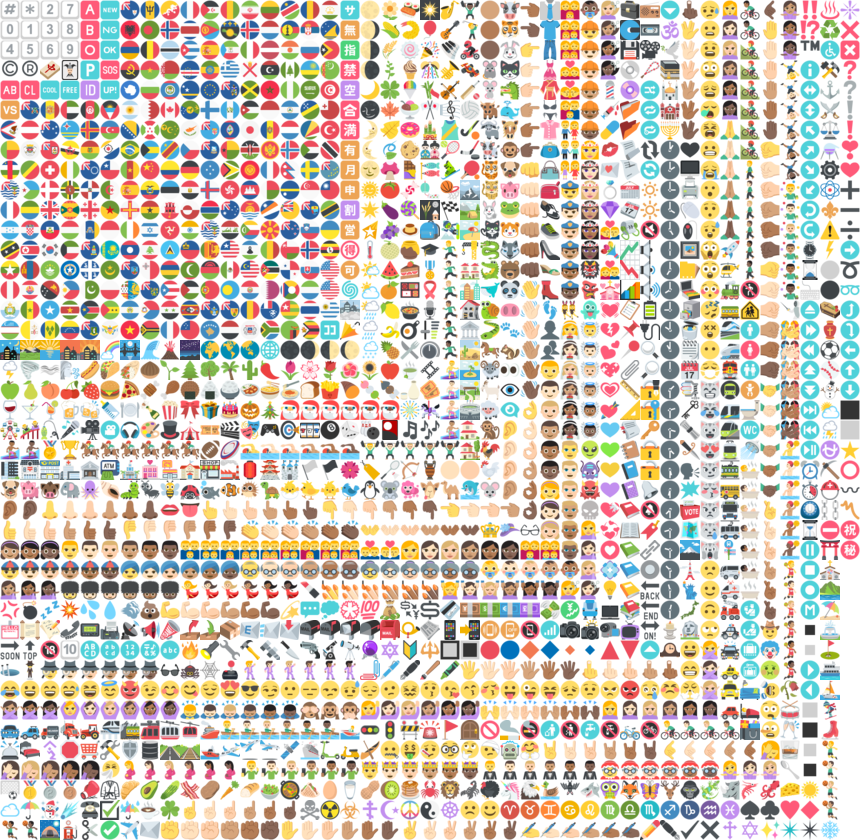
| W: | H:
| W: | H:
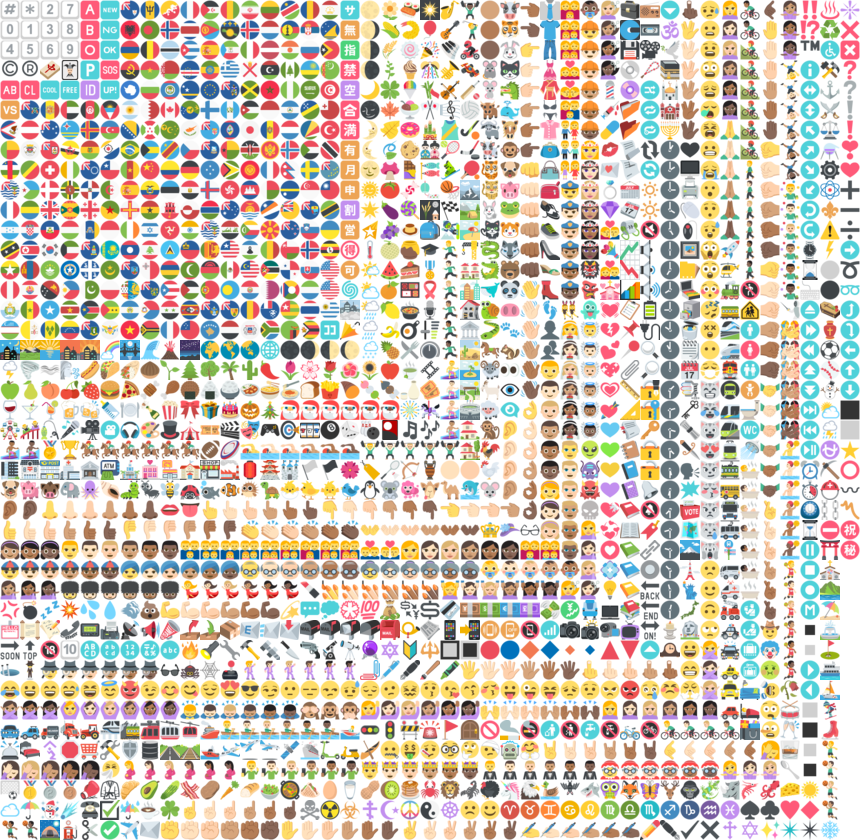
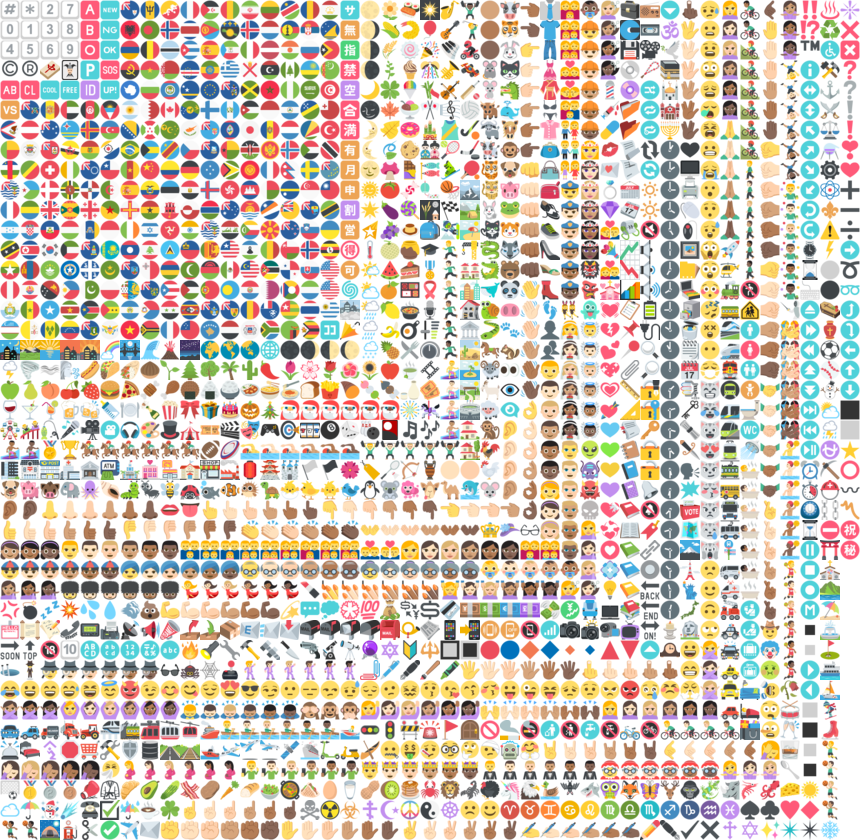
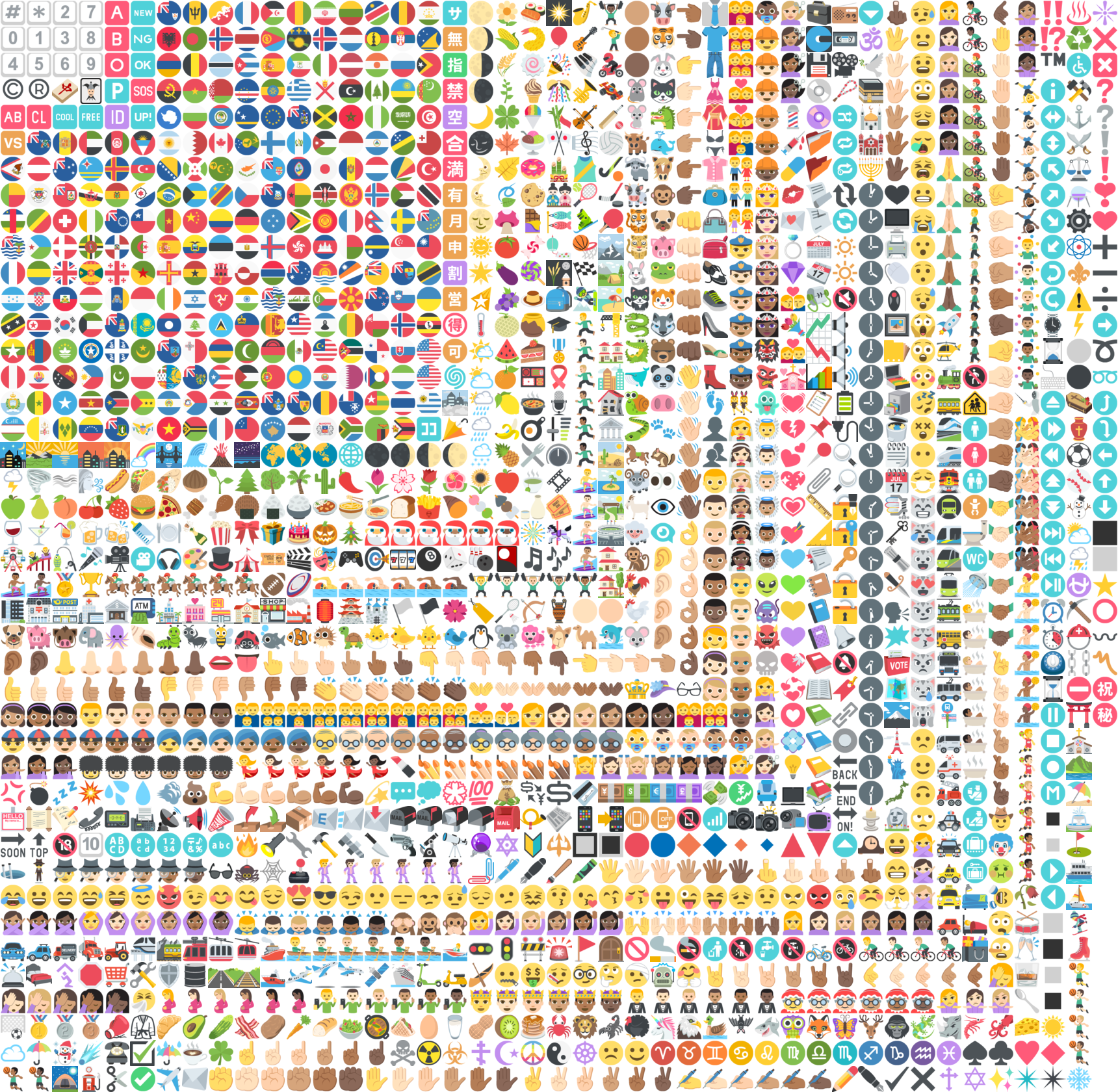
| W: | H:
| W: | H:
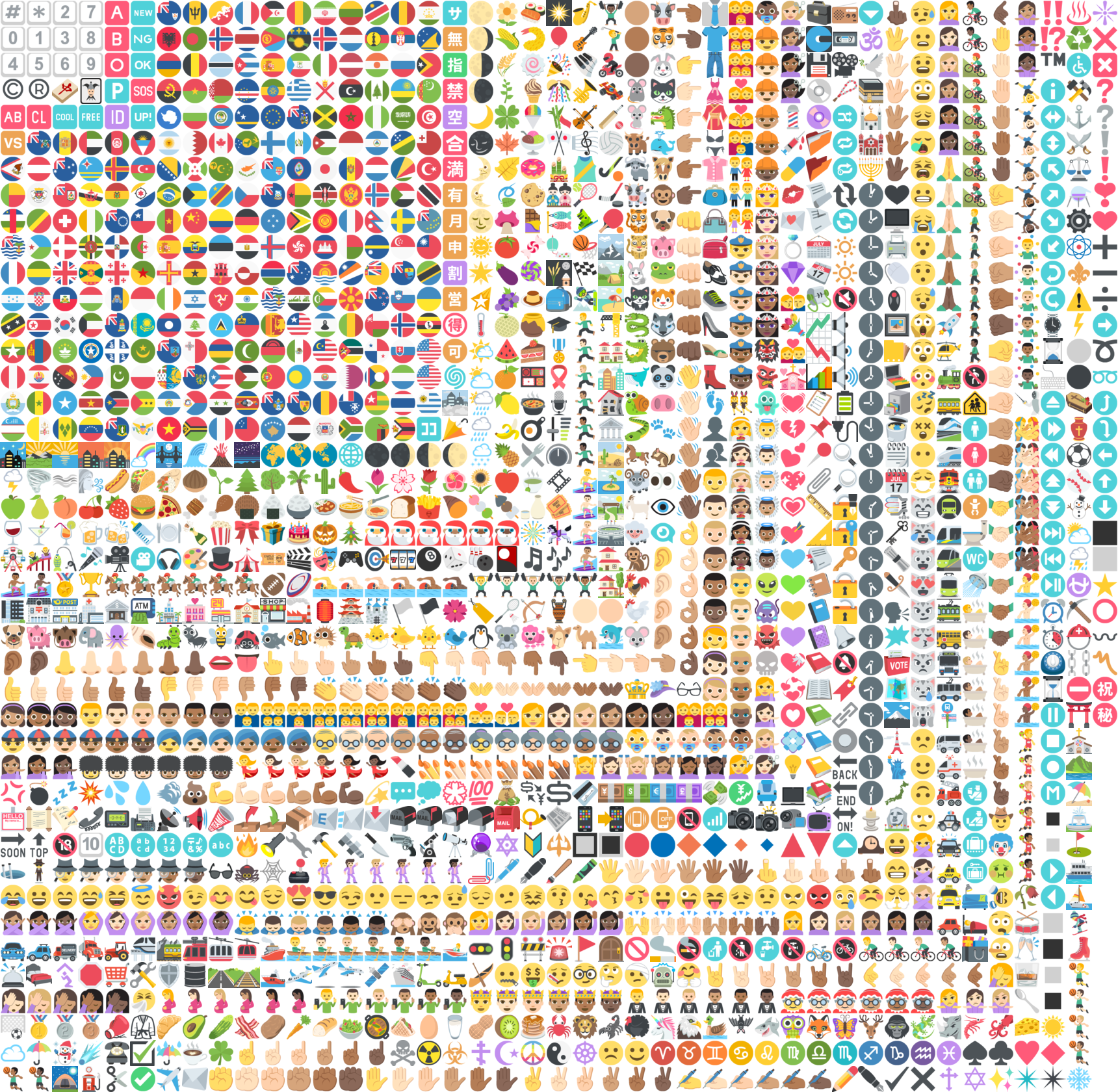
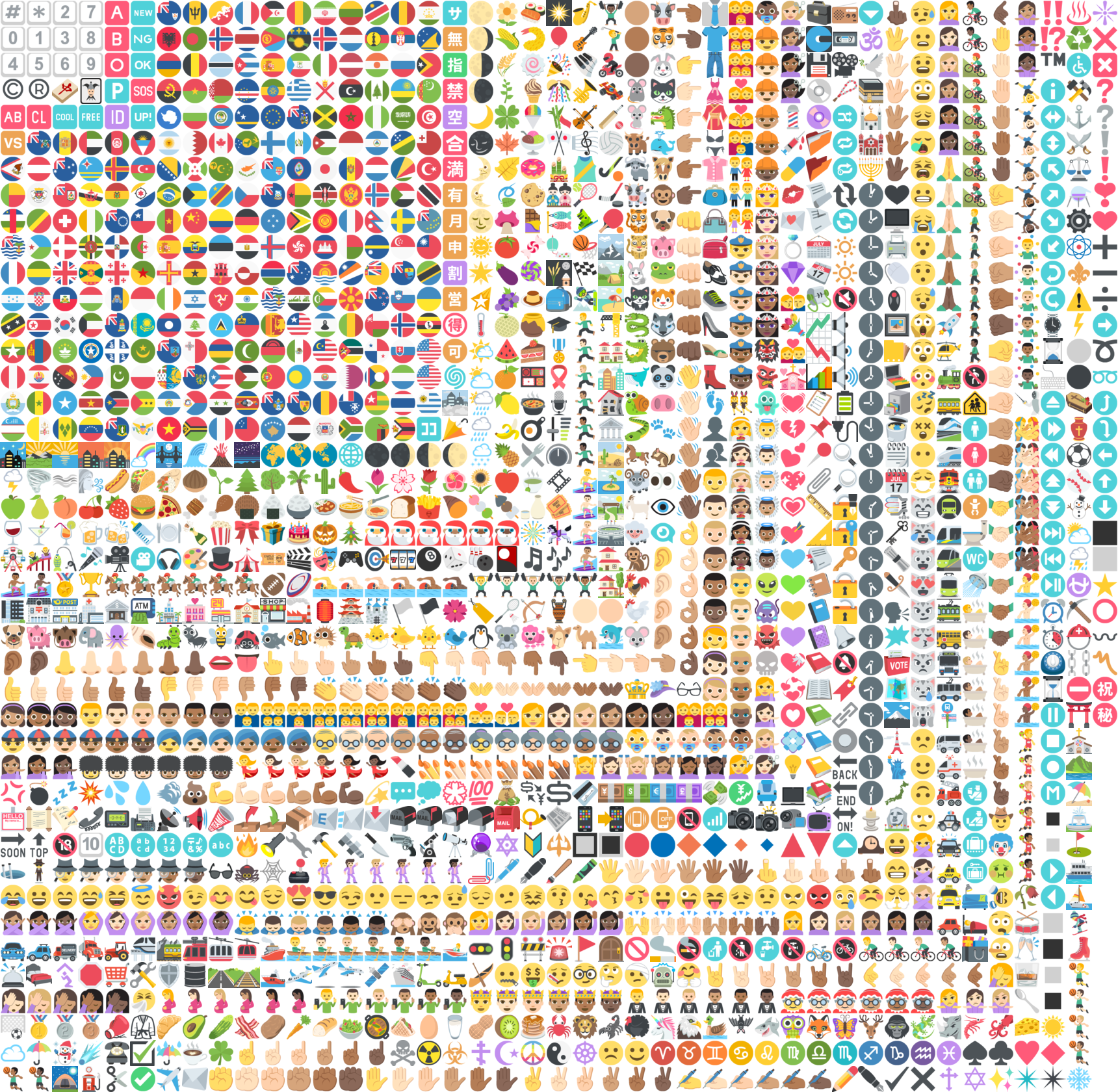
此差异已折叠。
app/helpers/emoji_helper.rb
0 → 100644
... | ... | @@ -18,7 +18,9 @@ |
"bootstrap-sass": "^3.3.6", | ||
"compression-webpack-plugin": "^0.3.2", | ||
"d3": "^3.5.11", | ||
"document-register-element": "^1.3.0", | ||
"dropzone": "^4.2.0", | ||
"emoji-unicode-version": "^0.2.1", | ||
"es6-promise": "^4.0.5", | ||
"jquery": "^2.2.1", | ||
"jquery-ujs": "^1.2.1", | ||
... | ... | @@ -29,6 +31,8 @@ |
"raw-loader": "^0.5.1", | ||
"select2": "3.5.2-browserify", | ||
"stats-webpack-plugin": "^0.4.3", | ||
"string.fromcodepoint": "^0.2.1", | ||
"string.prototype.codepointat": "^0.2.0", | ||
"timeago.js": "^2.0.5", | ||
"underscore": "^1.8.3", | ||
"vue": "^2.1.10", | ||
... | ... |
此差异已折叠。
spec/javascripts/gl_emoji_spec.js
0 → 100644