MMDLoader: Implement MMDToonMaterial. (#21922)
* Add prototype of MMDToonMaterial. * Try to use ShaderMaterial to simulate MeshToonMaterial. * Done simulate MeshToonMaterial with ShaderMaterial. * Clean up MMDToonMaterial. * Add envMap uniforms. * Enable merge gradient map with phong material but got warning. * Enable specular and shininess in MMDToonMaterial. * Done combining Phong/Toon/Matcap materials. * Pre PR chores. * fix typos. * Set color to diffuse uniform. * Update e2e test screenshots. * Set .shininess to original and cut half irradiance. * Remove dotNL from irradiance and update screenshots. * Fix with PR comments. * Modify maps file name storing position. * Clean extra code. * Update comment and use setter/getters to improve compatibility. * Simplify defineProterties with defineProterty. * Improve exposing property pattern. * Simplify defineProterties with defineProterty.
Showing
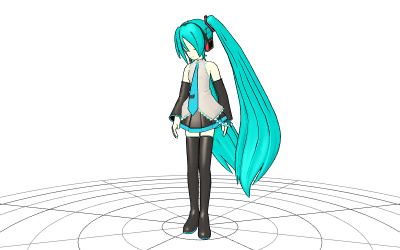
| W: | H:
| W: | H:
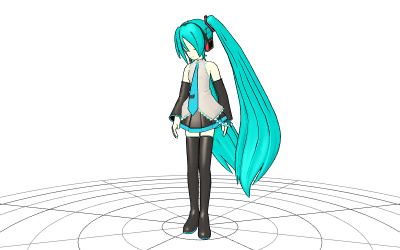
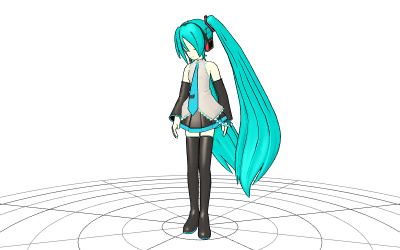