Skip to content
体验新版
项目
组织
正在加载...
登录
切换导航
打开侧边栏
Miykael_xxm
leetcode
提交
15be5efb
L
leetcode
项目概览
Miykael_xxm
/
leetcode
与 Fork 源项目一致
从无法访问的项目Fork
通知
1
Star
0
Fork
0
代码
文件
提交
分支
Tags
贡献者
分支图
Diff
Issue
0
列表
看板
标记
里程碑
合并请求
0
Wiki
0
Wiki
分析
仓库
DevOps
项目成员
Pages
L
leetcode
项目概览
项目概览
详情
发布
仓库
仓库
文件
提交
分支
标签
贡献者
分支图
比较
Issue
0
Issue
0
列表
看板
标记
里程碑
合并请求
0
合并请求
0
Pages
分析
分析
仓库分析
DevOps
Wiki
0
Wiki
成员
成员
收起侧边栏
关闭侧边栏
动态
分支图
创建新Issue
提交
Issue看板
前往新版Gitcode,体验更适合开发者的 AI 搜索 >>
提交
15be5efb
编写于
3月 24, 2020
作者:
L
lucifer
浏览文件
操作
浏览文件
下载
差异文件
Merge branch 'master' of
https://github.com/azl397985856/leetcode
上级
839b652f
93973a68
变更
2
隐藏空白更改
内联
并排
Showing
2 changed file
with
160 addition
and
51 deletion
+160
-51
problems/26.remove-duplicates-from-sorted-array.md
problems/26.remove-duplicates-from-sorted-array.md
+25
-1
problems/365.water-and-jug-problem.md
problems/365.water-and-jug-problem.md
+135
-50
未找到文件。
problems/26.remove-duplicates-from-sorted-array.md
浏览文件 @
15be5efb
...
@@ -64,9 +64,11 @@ for (int i = 0; i < len; i++) {
...
@@ -64,9 +64,11 @@ for (int i = 0; i < len; i++) {
-
如果是数据是无序的,就不可以用这种方式了,从这里也可以看出排序在算法中的基础性和重要性。
-
如果是数据是无序的,就不可以用这种方式了,从这里也可以看出排序在算法中的基础性和重要性。
-
注意nums为空时的边界条件。
## 代码
## 代码
*
语言支持:JS,Python
*
语言支持:JS,Python
,C++
Javascript Code:
Javascript Code:
```
js
```
js
...
@@ -76,6 +78,7 @@ Javascript Code:
...
@@ -76,6 +78,7 @@ Javascript Code:
*/
*/
var
removeDuplicates
=
function
(
nums
)
{
var
removeDuplicates
=
function
(
nums
)
{
const
size
=
nums
.
length
;
const
size
=
nums
.
length
;
if
(
size
==
0
)
return
0
;
let
slowP
=
0
;
let
slowP
=
0
;
for
(
let
fastP
=
0
;
fastP
<
size
;
fastP
++
)
{
for
(
let
fastP
=
0
;
fastP
<
size
;
fastP
++
)
{
if
(
nums
[
fastP
]
!==
nums
[
slowP
])
{
if
(
nums
[
fastP
]
!==
nums
[
slowP
])
{
...
@@ -101,3 +104,24 @@ class Solution:
...
@@ -101,3 +104,24 @@ class Solution:
else
:
else
:
return
0
return
0
```
```
C++ Code:
```
cpp
class
Solution
{
public:
int
removeDuplicates
(
vector
<
int
>&
nums
)
{
if
(
nums
.
empty
())
return
0
;
int
fast
,
slow
;
fast
=
slow
=
0
;
while
(
fast
!=
nums
.
size
()){
if
(
nums
[
fast
]
==
nums
[
slow
])
fast
++
;
else
{
slow
++
;
nums
[
slow
]
=
nums
[
fast
];
fast
++
;
}
}
return
slow
+
1
;
}
};
```
problems/365.water-and-jug-problem.md
浏览文件 @
15be5efb
...
@@ -25,7 +25,66 @@ Output: False
...
@@ -25,7 +25,66 @@ Output: False
```
```
## 思路
## BFS(超时)
### 思路
两个水壶的水我们考虑成状态,然后我们不断进行倒的操作,改变状态。那么初始状态就是(0 0) 目标状态就是 (any, z)或者 (z, any),其中any 指的是任意升水。
已题目的例子,其过程示意图,其中括号表示其是由哪个状态转移过来的:
0 0
3 5(0 0) 3 0 (0 0 )0 5(0 0)
3 2(0 5) 0 3(0 0)
0 2(3 2)
2 0(0 2)
2 5(2 0)
3 4(2 5) bingo
### 代码
```
python
class
Solution
:
def
canMeasureWater
(
self
,
x
:
int
,
y
:
int
,
z
:
int
)
->
bool
:
if
x
+
y
<
z
:
return
False
queue
=
[(
0
,
0
)]
seen
=
set
((
0
,
0
))
while
(
len
(
queue
)
>
0
):
a
,
b
=
queue
.
pop
(
0
)
if
a
==
z
or
b
==
z
or
a
+
b
==
z
:
return
True
states
=
set
()
states
.
add
((
x
,
b
))
states
.
add
((
a
,
y
))
states
.
add
((
0
,
b
))
states
.
add
((
a
,
0
))
states
.
add
((
min
(
x
,
b
+
a
),
0
if
b
<
x
-
a
else
b
-
(
x
-
a
)))
states
.
add
((
0
if
a
+
b
<
y
else
a
-
(
y
-
b
),
min
(
b
+
a
,
y
)))
for
state
in
states
:
if
state
in
seen
:
continue
;
queue
.
append
(
state
)
seen
.
add
(
state
)
return
False
```
**复杂度分析**
-
时间复杂度:由于状态最多有$O((x + 1)
* (y + 1))$ 种,因此总的时间复杂度为$O(x *
y)$。
-
空间复杂度:我们使用了队列来存储状态,set 存储已经访问的元素,空间复杂度和状态数目一致,因此空间复杂度是$O(x
*
y)$。
上面的思路很直观,但是很遗憾这个算法在 LeetCode 的表现是 TLE(Time Limit Exceeded)。不过如果你能在真实面试中写出这样的算法,我相信大多数情况是可以过关的。
我们来看一下有没有别的解法。实际上,上面的算法就是一个标准的 BFS。如果从更深层次去看这道题,会发现这道题其实是一道纯数学问题,类似的纯数学问题在 LeetCode 中也会有一些,不过大多数这种题目,我们仍然可以采取其他方式 AC。那么让我们来看一下如何用数学的方式来解这个题。
## 数学法 - 最大公约数
### 思路
这是一道关于
`数论`
的题目,确切地说是关于
`裴蜀定理`
(英语:Bézout's identity)的题目。
这是一道关于
`数论`
的题目,确切地说是关于
`裴蜀定理`
(英语:Bézout's identity)的题目。
...
@@ -40,60 +99,61 @@ ax+by=m
...
@@ -40,60 +99,61 @@ ax+by=m
```
```
因此这道题可以完全转化为
`裴蜀定理`
。
因此这道题可以完全转化为
`裴蜀定理`
。还是以题目给的例子
`x = 3, y = 5, z = 4`
,我们其实可以表示成
`3 * 3 - 1 * 5 = 4`
, 即
`3 * x - 1 * y = z`
。我们用a和b分别表示3
升的水壶和5升的水壶。那么我们可以:
## 关键点解析
-
数论
-
倒满a(
**1**
)
-
裴蜀定理
-
将a倒到b
-
再次倒满a(
**2**
)
-
再次将a倒到b(a这个时候还剩下1升)
-
倒空b(
**-1**
)
-
将剩下的1升倒到b
-
将a倒满(
**3**
)
-
将a倒到b
-
b此时正好是4升
## 代码
上面的过程就是
`3 * x - 1 * y = z`
的具体过程解释。
```
js
**也就是说我们只需要求出x和y的最大公约数d,并判断z是否是d的整数倍即可。**
/*
* @lc app=leetcode id=365 lang=javascript
### 代码
*
* [365] Water and Jug Problem
代码支持:Python3,JavaScript
*
* https://leetcode.com/problems/water-and-jug-problem/description/
*
Python Code:
* algorithms
* Medium (28.76%)
```
python
* Total Accepted: 27K
class
Solution
:
* Total Submissions: 93.7K
def
canMeasureWater
(
self
,
x
:
int
,
y
:
int
,
z
:
int
)
->
bool
:
* Testcase Example: '3\n5\n4'
if
x
+
y
<
z
:
*
return
False
* You are given two jugs with capacities x and y litres. There is an infinite
* amount of water supply available. You need to determine whether it is
if
(
z
==
0
):
* possible to measure exactly z litres using these two jugs.
return
True
*
* If z liters of water is measurable, you must have z liters of water
if
(
x
==
0
):
* contained within one or both buckets by the end.
return
y
==
z
*
* Operations allowed:
if
(
y
==
0
):
*
return
x
==
z
*
* Fill any of the jugs completely with water.
def
GCD
(
a
,
b
):
* Empty any of the jugs.
smaller
=
min
(
a
,
b
)
* Pour water from one jug into another till the other jug is completely full
while
smaller
:
* or the first jug itself is empty.
if
a
%
smaller
==
0
and
b
%
smaller
==
0
:
*
return
smaller
*
smaller
-=
1
* Example 1: (From the famous "Die Hard" example)
*
return
z
%
GCD
(
x
,
y
)
==
0
*
```
* Input: x = 3, y = 5, z = 4
* Output: True
JavaScript:
*
*
* Example 2:
```
js
*
*
* Input: x = 2, y = 6, z = 5
* Output: False
*
*/
/**
/**
* @param {number} x
* @param {number} x
* @param {number} y
* @param {number} y
...
@@ -121,3 +181,28 @@ var canMeasureWater = function(x, y, z) {
...
@@ -121,3 +181,28 @@ var canMeasureWater = function(x, y, z) {
return
z
%
GCD
(
x
,
y
)
===
0
;
return
z
%
GCD
(
x
,
y
)
===
0
;
};
};
```
```
实际上求最大公约数还有更好的方式,比如辗转相除法:
```
python
def
GCD
(
a
,
b
):
if
b
==
0
:
return
a
return
GCD
(
b
,
a
%
b
)
```
**复杂度分析**
-
时间复杂度:$O(log(max(a, b)))$
-
空间复杂度:空间复杂度取决于递归的深度,因此空间复杂度为 $O(log(max(a, b)))$
## 关键点分析
-
数论
-
裴蜀定理
更多题解可以访问我的LeetCode题解仓库:https://github.com/azl397985856/leetcode 。 目前已经接近30K star啦。
大家也可以关注我的公众号《脑洞前端》获取更多更新鲜的LeetCode题解
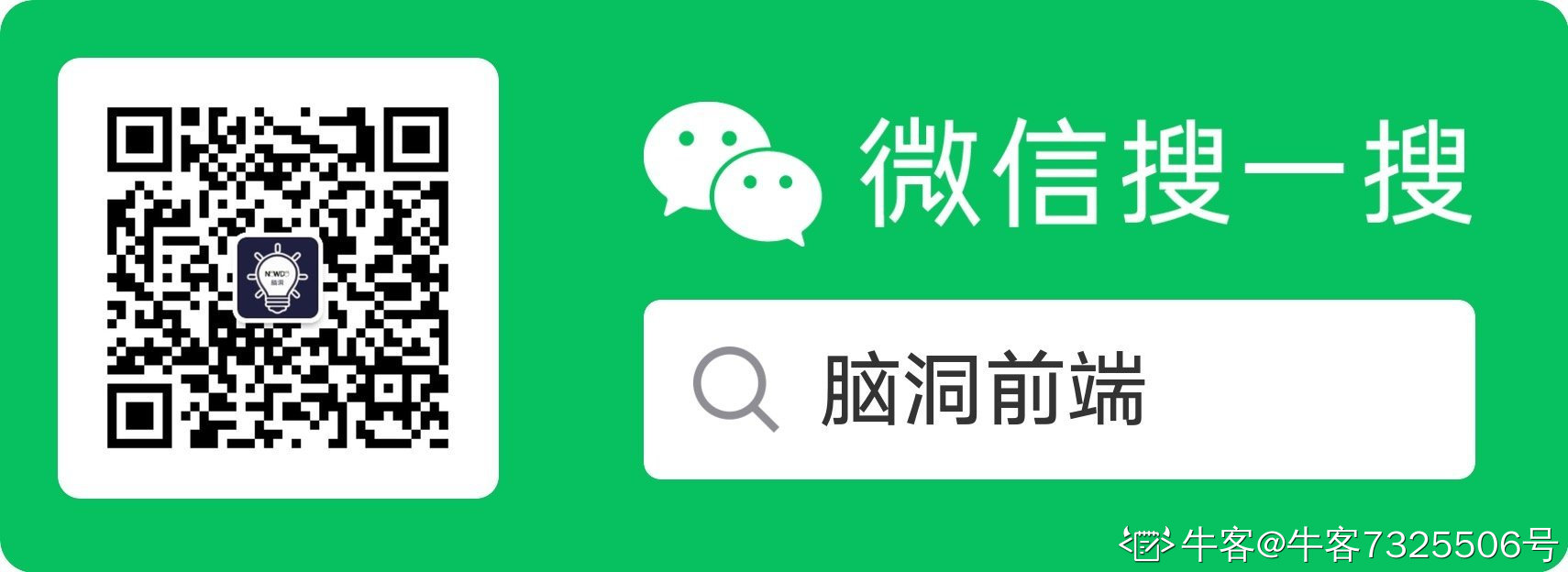
编辑
预览
Markdown
is supported
0%
请重试
或
添加新附件
.
添加附件
取消
You are about to add
0
people
to the discussion. Proceed with caution.
先完成此消息的编辑!
取消
想要评论请
注册
或
登录